在数字化转型加速的今天,各行各业都在寻求利用最新技术提升用户体验、增强用户粘性。作为曲靖本土媒体的先锋,曲靖M最近推出了一款全新的评论互动抽奖模块,该模块依托先进的人工智能技术开发。
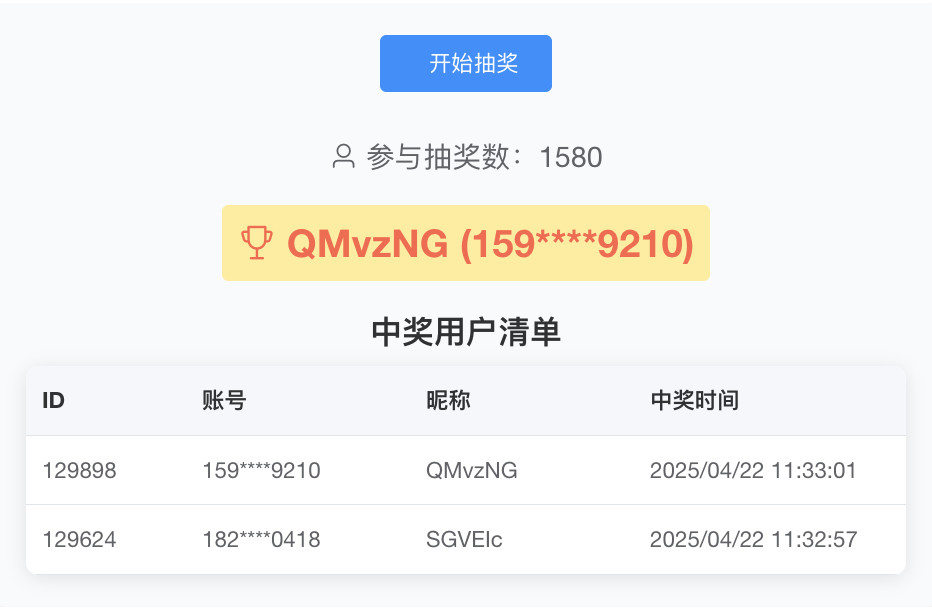
以下代码段展示了如何通过与AI进行对话式互动来实现的最终开发成果。这种方式不仅促进了开发者与AI之间的紧密协作,还极大地提高了开发效率和灵活性。通过这种交互模式,开发者能够快速迭代原型、测试新想法,并根据实时反馈进行调整优化。此外,它还支持自然语言处理功能,允许开发者以更直观的方式描述需求,使得编码过程更加流畅高效,同时也降低了技术门槛,使更多非技术人员也能参与到开发过程中来。
<template>
<div class="participation-award">
<el-button
ref="startLotteryButton"
type="primary"
:loading="isLoading"
:disabled="disabled"
icon="el-icon-dice"
@click="startLottery"
>
{{ lotteryButtonText }}
</el-button>
<div v-show="commenterCount > 0" class="participation-award-count">
<i class="el-icon-user" /> 参与抽奖数:{{ commenterCount }}
</div>
<div class="lottery-draw">
<div v-if="currentNickname" :class="{ 'current-nickname': isWinnerDisplayed }">
<i class="el-icon-trophy" /> {{ currentNickname }}
</div>
</div>
<div class="winners-list">
<h3>中奖用户清单</h3>
<el-table :data="winnersList" style="width: 100%" :header-cell-style="{ background: '#f5f7fa', color: '#303133' }">
<el-table-column prop="userid" label="ID" width="100" />
<el-table-column label="账号">
<template slot-scope="scope">
{{ maskPhoneNumber(scope.row.account) }}
</template>
</el-table-column>
<el-table-column prop="nickname" label="昵称" />
<el-table-column prop="winningTime" label="中奖时间" width="170" />
</el-table>
</div>
</div>
</template>
<script>
import { getCommenterList } from '@/api/comment'
import { maskPhoneNumber } from '@/utils/index'
const defaultLotteryButtonText = '开始抽奖'
export default {
name: 'ParticipationAward',
props: {
postId: {
type: Number,
default: 0
}
},
data() {
return {
lotteryButtonText: defaultLotteryButtonText,
commenterCount: 0,
commenterList: [],
currentNickname: '',
intervalId: null,
isWinnerDisplayed: false,
winnersList: [], // 存储每次中奖的用户信息
isLoading: false, // 控制按钮的加载状态
disabled: false
}
},
methods: {
async startLottery() {
if (this.isLoading) return // 如果已经在加载中,直接返回
this.disabled = true
// 先获取最新的参与者数据
try {
this.commenterCount = 0
this.commenterList = []
this.isLoading = true
this.lotteryButtonText = '获取最新参与者数据'
const { data } = await getCommenterList(this.postId)
this.commenterList = data
this.commenterCount = this.commenterList.length
if (this.commenterCount === 0) {
this.$message.error('没有参与者')
this.isLoading = false
this.disabled = false
this.lotteryButtonText = defaultLotteryButtonText
return
}
this.isLoading = false
this.lotteryButtonText = defaultLotteryButtonText
// 设置按钮为正在抽奖状态
this.lotteryButtonText = '正在抽奖中.....'
// 重置闪烁效果状态
this.isWinnerDisplayed = false
// 开始动态显示昵称和账号
this.intervalId = setInterval(() => {
const randomIndex = Math.floor(Math.random() * this.commenterList.length)
const randomCommenter = this.commenterList[randomIndex]
this.currentNickname = `${randomCommenter.nickname} (${this.maskPhoneNumber(randomCommenter.account)})`
}, 100)
// 停止动态显示昵称并选择中奖者
setTimeout(() => {
clearInterval(this.intervalId)
const winnerIndex = Math.floor(Math.random() * this.commenterList.length)
const winner = this.commenterList[winnerIndex]
this.currentNickname = `${winner.nickname} (${this.maskPhoneNumber(winner.account)})`
this.isWinnerDisplayed = true // 显示中奖者时应用闪烁效果
// 获取当前时间并格式化
const winningTime = new Date().toLocaleString('zh-CN', {
year: 'numeric',
month: '2-digit',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
second: '2-digit',
hour12: false
})
// 添加中奖者到中奖用户清单
this.winnersList.unshift({
userid: winner.userid,
account: this.maskPhoneNumber(winner.account),
nickname: winner.nickname,
winningTime: winningTime
})
// 恢复按钮状态
this.isLoading = false
this.disabled = false
this.lotteryButtonText = defaultLotteryButtonText
}, 3000)
} catch (error) {
this.$message.error('获取参与者数据失败,请重试')
console.error('获取参与者数据失败:', error)
this.isLoading = false
this.disabled = false
}
},
maskPhoneNumber(phoneNumber) {
return maskPhoneNumber(phoneNumber)
}
}
}
</script>
<style lang="scss" scoped>
.participation-award {
background-color: #f9fafc;
padding: 20px;
border-radius: 8px;
box-shadow: 0 2px 12px 0 rgba(0, 0, 0, 0.1);
}
.el-button {
margin-bottom: 20px;
}
.participation-award-count {
margin-top: 10px;
font-size: 18px;
color: #606266;
}
.lottery-draw {
margin-top: 20px;
font-size: 24px;
font-weight: bold;
color: #ff6347;
}
.current-nickname {
animation: blink 1s step-start infinite;
background-color: #ffec99;
padding: 10px;
border-radius: 4px;
display: inline-block;
}
@keyframes blink {
50% {
opacity: 0;
}
}
.winners-list {
margin-top: 20px;
}
.winners-list h3 {
margin-bottom: 10px;
font-size: 20px;
font-weight: bold;
color: #303133;
}
.el-table {
border-radius: 8px;
overflow: hidden;
box-shadow: 0 2px 12px 0 rgba(0, 0, 0, 0.1);
}
.el-table::before {
height: 0;
}
.el-table th {
background-color: #f5f7fa;
color: #303133;
}
</style>
+1